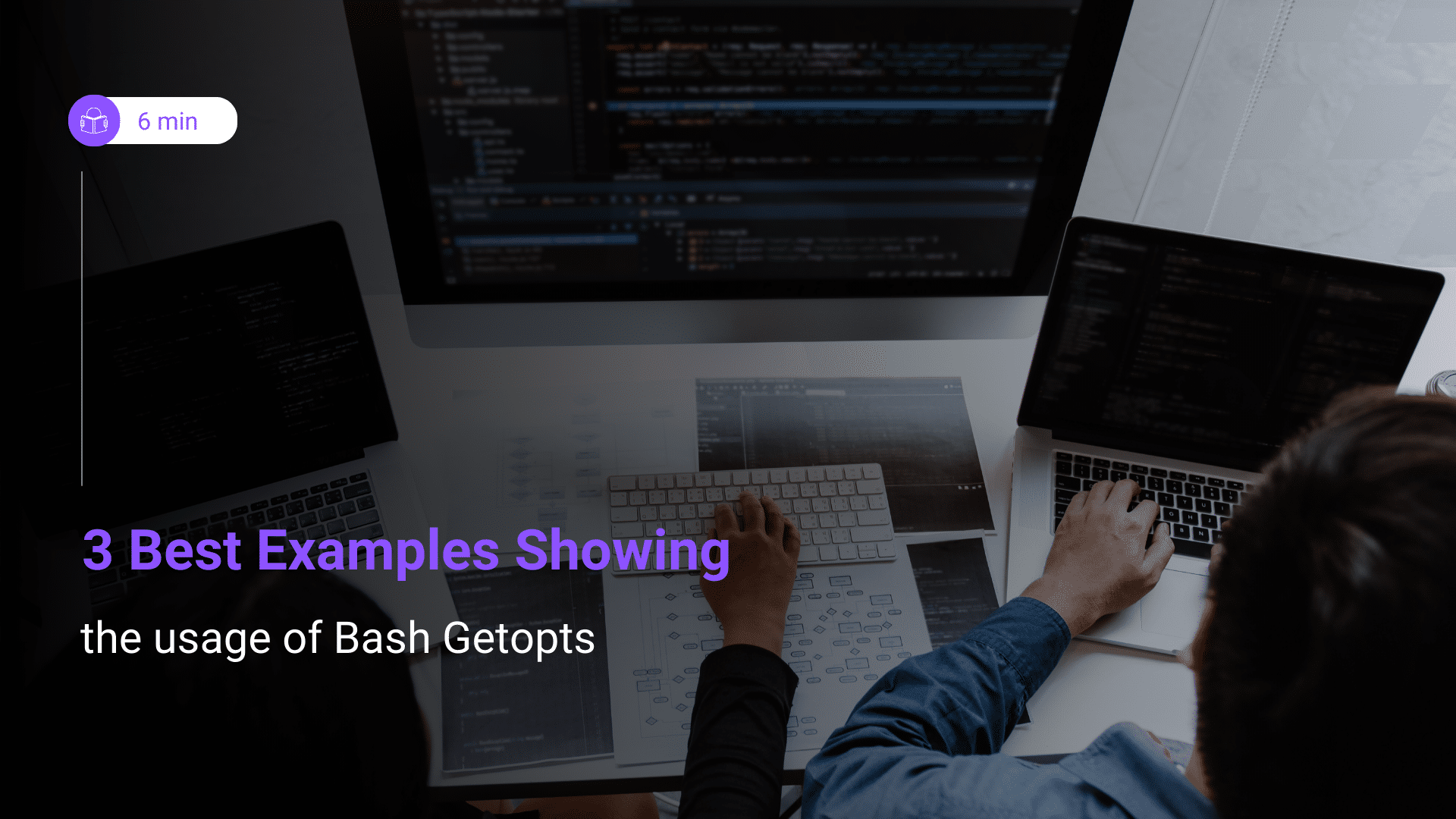
The Bash getopts command is a useful tool that permits you to parse command-line options in your shell scripts. It provides a best way to control the behaviour of your script and handle user input on the basis of specified options. In this article we’ll delve into bash getopts and will know some best examples of bash getopts. But before diving into the practical example, it is crucial to understand the basics of how bash getopts works.
What is Getopts?
Getopts is the command that comes with Bash. It is specifically designed to help you in managing the command line options as well as arguments as per the provided script or function.
In short words, it helps in ‘decoding’ user input and finding what possible actions the script should take.
If we compare Getopts to positional parameters, getopts is considered less prone to errors and more reliable. This is due to getopts permitting options and arguments to be clearly defined and labeled, that will eventually help in reducing the confusion and any mistakes that can be caused from the strict order needs of positional parameters.
Before we dive further into getopts example, it is important to understand the two important terms that we will frequently use in this article: options and arguments.
1. Options: Options, also known as flags, are special types of positional parameters that start with a hyphen (-) and change the behavior of the command or the script as per need. They are typically single letters, such as -v or -h.
2. Arguments: Arguments, often called parameters. They are positional parameters that provide you with specific data to the command or script. They might follow any option if that option needs further details, or they could also stand alone as inputs to the script or command itself.
Using getopts: Step by Step Guide
1. Initialize Variables:Â
  suffix=".bak"   verbose=0   ```
Here, we set default values for the variables `suffix` and `verbose`.
2. Parse Command Line Options:
  while getopts ":vs:" opt; do    case ${opt} in     v) verbose=1;;     s) suffix="$OPTARG";;     \?) echo "Invalid option: -$OPTARG" >&2;;    esac   done   shift $((OPTIND -1))   ```
- We use `getopts` to parse the command-line options.
- `-v` is a flag for verbose mode, while `-s` takes an argument for a custom suffix.
- Inside the loop, we handle each option accordingly.
- `shift $((OPTIND -1))` removes the options that have been processed from the positional parameters.
3. Main Script:
  for file in "$@"; do    if [ $verbose -eq 1 ]; then     echo "Processing $file..."    fi    cp "$file" "$file$suffix"   done   ```
- The main script iterates over each file provided as an argument.
- If verbose mode is enabled, it prints a message indicating which file is being processed.
- It creates a backup file for each input file with the specified suffix using `cp`.
4. Running the Script:
  ./backup.sh -vs ".old" file1.txt file2.txt   ```
This command executes the script with verbose mode enabled (`-v`), a custom suffix of `.old` (`-s “.old”`), and two input files: `file1.txt` and `file2.txt`.
5. Output:
The script creates backup files `file1.txt.old` and `file2.txt.old` in the same directory as the original files.
If verbose mode is enabled, it also prints messages indicating the processing of each file.
Using getopts: Examples
Bash `getopts` is a built-in command used to parse command-line options. It’s great for handling flags and parameters in shell scripts. Here are three simple example:
Example 1:Â
while getopts ":a:b:" opt; do  case $opt in   a) echo "Option a with argument: $OPTARG";;   b) echo "Option b with argument: $OPTARG";;   \?) echo "Invalid option: -$OPTARG";;  esac done
In this script:
– `getopts` sets up the option parsing.
– The string `”ab:”` specifies that `a` and `b` options can take arguments.
– Inside the `while` loop, `getopts` iterates over each option passed.
– `case` statement handles different options.
– `OPTARG` holds the value of the argument for the current option.
To run this script, say you name it `example.sh`, run:
./example.sh -a valueA -b valueB
This would output:
Option a with argument: valueA Option b with argument: valueB
Remember:
– Use `:` after each option letter to indicate it requires an argument.
– Precede the option string with a colon if you want to handle errors yourself.
– Use `OPTARG` to access the value of an option’s argument.
– Loop through options using `while getopts`.
– Handle each option using a `case` statement.
Example 2:Â
#!/bin/bash # Initialize variables with default values verbose=0 name="World" # Parse command line options while getopts ":vn:" opt; do  case ${opt} in   v) verbose=1;;   n) name="$OPTARG";;   \?) echo "Invalid option: -$OPTARG" >&2;;  esac done shift $((OPTIND -1)) # Main script if [ $verbose -eq 1 ]; then  echo "Hello, $name!" else  echo "Hello!" fi
In this script:
– We define two options: `-v` for verbose mode and `-n` to specify a name.
– `v` is a flag, so it doesn’t take an argument, while `n` does take an argument, which is stored in `OPTARG`.
– We set default values for the variables `verbose` and `name`.
– We use `getopts` to parse the command-line options.
– Inside the `while` loop, we handle each option accordingly.
– We shift the positional parameters to remove the options that have been processed.
– Finally, we use the parsed options to execute the main script logic, which in this case is printing a greeting message.
To run this script, suppose you save it as `greet.sh`, you can execute it like this:
./greet.sh -v -n Alice
This would output:
Hello, Alice!
Example 3:
#!/bin/bash # Initialize variables with default values suffix=".bak" verbose=0 # Parse command line options while getopts ":vs:" opt; do  case ${opt} in   v) verbose=1;;   s) suffix="$OPTARG";;   \?) echo "Invalid option: -$OPTARG" >&2;;  esac done shift $((OPTIND -1)) # Main script for file in "$@"; do  if [ $verbose -eq 1 ]; then   echo "Processing $file..."  fi  cp "$file" "$file$suffix" done
In this script:
– We define two options: `-v` for verbose mode and `-s` to specify a custom suffix for backup files.
– `v` is a flag, while `s` takes an argument, which is stored in `OPTARG`.
– We set default values for the variables `suffix` and `verbose`.
– `getopts` parses the command-line options.
– Inside the loop, we handle each option accordingly.
– We shift the positional parameters to remove the options that have been processed.
– The main script iterates over each file provided as an argument and creates a backup file with the specified suffix.
To run this script, suppose you save it as `backup.sh`, you can execute it like this:
./backup.sh -vs ".old" file1.txt file2.txt
This would create backup files `file1.txt.old` and `file2.txt.old` and print verbose messages about processing each file.
Conclusion
In conclusion, this article helps you by providing the best example of using Getopts and we also learned about the getopts command. Getopts is the command that comes with Bash. It is specifically designed to help you in managing the command line options as well as arguments as per the provided script or function.