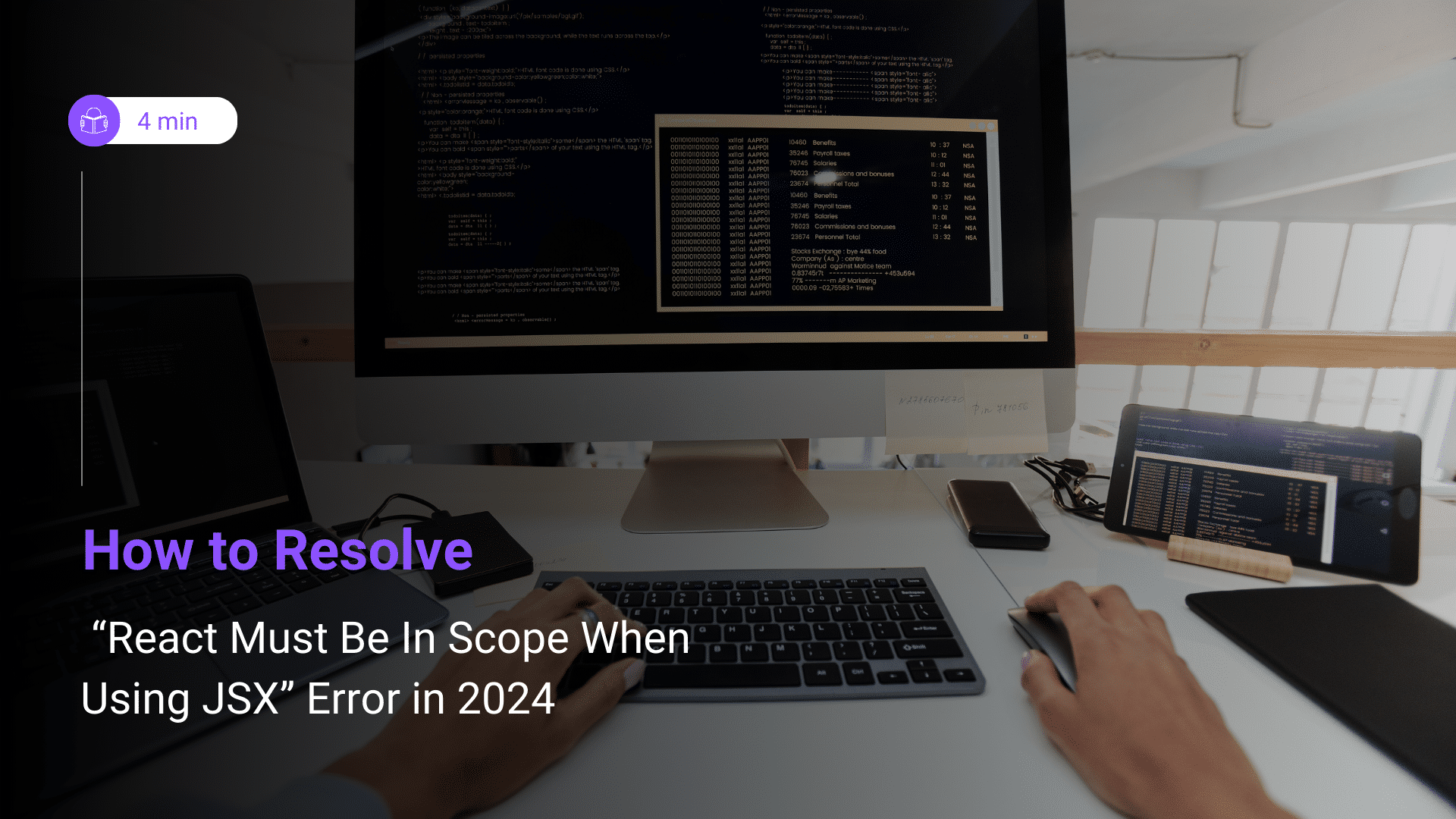
What is this“React Must Be In Scope When Using JSX” Error?
Sometimes working with React can be complex for you, specifically when you are dealing with errors that are not always easy to know.
A common error you may face is this “React must be in scope when using JSX” error. This error happens when the React is not imported properly or initialized in the component that uses JSX syntax. Now let’s see why you are getting this error!
Causes of Getting This “React Must Be In Scope When Using JSX” Error
Before getting knowledge about the error, you must know about JSX syntax. JSX is a syntax extension that permits you to write HTML code in JavaScript. The causes of getting this “react must be in scope when using JSX” error are listed below:
1. Missing React import: For JSX syntax to work, React needs to be imported into your file.
// Incorrect function MyComponent() { return ( <div>Hello, World!</div> ); }
// Correct import React from 'react'; function MyComponent() { return ( <div>Hello, World!</div> ); }
2. Incorrect file extension: Make sure your file has a .jsx extension, or configure your project to support JSX in .js files. If you still encounter the error, it might be a configuration issue.
3. Incorrect JSX syntax: Double-check your JSX syntax and make sure it’s correct and doesn’t contain any syntax errors. For instance:
// Incorrect - missing closing tag function MyComponent() { return ( <div>Hello, World!</div> {/* Missing closing tag */} ); }
// Correct function MyComponent() { return ( <div>Hello, World!</div> ); }
7 Ways to Resolve the “react must be in scope when using JSX” Error
1. Import React in every JSX file:
Ensure that React is imported at the top of every file where JSX syntax is used.
// File: MyComponent.jsx import React from 'react'; function MyComponent() { return ( <div>Hello, World!</div> ); } export default MyComponent;
2. Use the correct file extension:
Ensure that your JSX files have the `.jsx` extension, or configure your project to support JSX in `.js` files.
// File: MyComponent.jsx import React from 'react'; function MyComponent() { return ( <div>Hello, World!</div> ); } export default MyComponent;
3. Check React installation:
Make sure React is installed in your project dependencies using npm or yarn.
npm install react
or
yarn add react
4. Provide correct path to React:
Ensure that the path to React in your import statement is correct.
// Incorrect import path import React from './node_modules/react';
// Correct import path import React from 'react';
5. Check build configurations:
If you’re using custom build configurations, ensure that they properly support JSX syntax and include React.
// webpack.config.js const path = require('path'); module.exports = { entry: './src/index.js', output: { path: path.resolve(__dirname, 'dist'), filename: 'bundle.js', }, module: { rules: [ { test: /\.(js|jsx)$/, exclude: /node_modules/, use: { loader: 'babel-loader', options: { presets: ['@babel/preset-env', '@babel/preset-react'], }, }, }, ], }, };
6. Check ESLint configuration:
If you’re using ESLint, ensure that it’s configured to recognize JSX syntax and React.
// .eslintrc.js module.exports = { parser: '@babel/eslint-parser', parserOptions: { ecmaVersion: 2021, sourceType: 'module', ecmaFeatures: { jsx: true, }, }, extends: ['eslint:recommended', 'plugin:react/recommended'], plugins: ['react'], rules: { // Add any specific ESLint rules here }, };
7. Restart development server:
After making changes, restart your development server to apply the updates.
These are some of the ways to resolve “react must be in scope when using JSX” error.
Conclusion
In conclusion, these are some of the ways, that might help you in resolving the “react must be in scope when using JSX” error. You can also resolve this issue by getting the help of the React Engineer team to fix it as soon as possible.
Frequently Asked Questions (FAQs)
1. What is JSX in react?
Answer: JSX stands for JavaScript XML, and allows you to write HTML code in React. This makes it easy to write and add the HTML code in React.
2. Is it possible to use JSX without React?
Answer: Yes it is possible to use JSX without React. JSX is necessary for using React. In fact, React without JSX is much easier. Every JSX element is the syntactic sugar to call React.
3. Where can I Put my JSX files?
Answer: Keep the JSX files in the Presets > Scripts folder.
4. What are the reasons for getting this error?
Answer: There can be many reasons like missing React import, incorrect file extension, and incorrect JSX syntax.